WiFi ESP32-CAM Controller on Android app..
https://play.google.com/store/apps/deta ... n_US&gl=US
Code: Select all
/*********
Rui Santos
Complete project details at https://RandomNerdTutorials.com/esp32-cam-video-streaming-web-server-camera-home-assistant/
IMPORTANT!!!
- Select Board "AI Thinker ESP32-CAM"
- GPIO 0 must be connected to GND to upload a sketch
- After connecting GPIO 0 to GND, press the ESP32-CAM on-board RESET button to put your board in flashing mode
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files.
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
*********/
/* Modified by Francisco Vásquez
* - Configured to use SoftAP instead of connecting to a wi-fi network.
* - Added TCP server (PORT 50000) to core 0 for managing commands.
* - Reduced image resolution to increase framerate.
* - Removed other boards code and left only AI thinker for code simplicity.
*/
#include <Ps3Controller.h>
#include "esp_camera.h"
#include <WiFi.h>
#include "esp_timer.h"
#include "img_converters.h"
#include "Arduino.h"
#include "fb_gfx.h"
#include "soc/soc.h" //disable brownout problems
#include "soc/rtc_cntl_reg.h" //disable brownout problems
#include "esp_http_server.h"
#include <HardwareSerial.h>
// SoftAP fixed ip address config.
IPAddress apIP = IPAddress(192, 168, 0, 13);
IPAddress ip;
// Tcp server port config.
TaskHandle_t tcpHandle;
WiFiServer server(400);
WiFiClient RemoteClient;
#define PART_BOUNDARY "123456789000000000000987654321"
#define PWDN_GPIO_NUM 32
#define RESET_GPIO_NUM -1
#define XCLK_GPIO_NUM 0
#define SIOD_GPIO_NUM 26
#define SIOC_GPIO_NUM 27
#define Y9_GPIO_NUM 35
#define Y8_GPIO_NUM 34
#define Y7_GPIO_NUM 39
#define Y6_GPIO_NUM 36
#define Y5_GPIO_NUM 21
#define Y4_GPIO_NUM 19
#define Y3_GPIO_NUM 18
#define Y2_GPIO_NUM 5
#define VSYNC_GPIO_NUM 25
#define HREF_GPIO_NUM 23
#define PCLK_GPIO_NUM 22
static const char* _STREAM_CONTENT_TYPE = "multipart/x-mixed-replace;boundary=" PART_BOUNDARY;
static const char* _STREAM_BOUNDARY = "\r\n--" PART_BOUNDARY "\r\n";
static const char* _STREAM_PART = "Content-Type: image/jpeg\r\nContent-Length: %u\r\n\r\n";
httpd_handle_t stream_httpd = NULL;
static esp_err_t stream_handler(httpd_req_t *req){
camera_fb_t * fb = NULL;
esp_err_t res = ESP_OK;
size_t _jpg_buf_len = 0;
uint8_t * _jpg_buf = NULL;
char * part_buf[64];
res = httpd_resp_set_type(req, _STREAM_CONTENT_TYPE);
if(res != ESP_OK){
return res;
}
while(true){
fb = esp_camera_fb_get();
if (!fb) {
res = ESP_FAIL;
} else {
if(fb->width > 400){
if(fb->format != PIXFORMAT_JPEG){
bool jpeg_converted = frame2jpg(fb, 80, &_jpg_buf, &_jpg_buf_len);
esp_camera_fb_return(fb);
fb = NULL;
if(!jpeg_converted){
res = ESP_FAIL;
}
} else {
_jpg_buf_len = fb->len;
_jpg_buf = fb->buf;
}
}
}
if(res == ESP_OK){
size_t hlen = snprintf((char *)part_buf, 64, _STREAM_PART, _jpg_buf_len);
res = httpd_resp_send_chunk(req, (const char *)part_buf, hlen);
}
if(res == ESP_OK){
res = httpd_resp_send_chunk(req, (const char *)_jpg_buf, _jpg_buf_len);
}
if(res == ESP_OK){
res = httpd_resp_send_chunk(req, _STREAM_BOUNDARY, strlen(_STREAM_BOUNDARY));
}
if(fb){
esp_camera_fb_return(fb);
fb = NULL;
_jpg_buf = NULL;
} else if(_jpg_buf){
free(_jpg_buf);
_jpg_buf = NULL;
}
if(res != ESP_OK){
break;
}
}
return res;
}
void startCameraServer(){
httpd_config_t config = HTTPD_DEFAULT_CONFIG();
config.server_port = 80;
httpd_uri_t index_uri = {
.uri = "/",
.method = HTTP_GET,
.handler = stream_handler,
.user_ctx = NULL
};
if (httpd_start(&stream_httpd, &config) == ESP_OK) {
httpd_register_uri_handler(stream_httpd, &index_uri);
}
}
// TCP server task
void tcpCore(void *algo) {
delay(5000);
server.begin();
for (;;) {
CheckForConnections();
uint8_t ReceiveBuffer[20];
while (RemoteClient.connected() && RemoteClient.available())
{
int Received = RemoteClient.read(ReceiveBuffer, sizeof(ReceiveBuffer));
Serial.write(ReceiveBuffer, Received);
Serial.println();
}
delay(10);
}
}
// Tcp server connection handler.
void CheckForConnections()
{
if (server.hasClient())
{
// If we are already connected to another computer,
// then reject the new connection. Otherwise accept
// the connection.
if (RemoteClient.connected())
{
Serial.println("Connection rejected");
server.available().stop();
}
else
{
Serial.write("play?");
RemoteClient = server.available();
}
}
}
void setup() {
WRITE_PERI_REG(RTC_CNTL_BROWN_OUT_REG, 0); //disable brownout detector
Serial.begin(115200);
Serial.setDebugOutput(true);
camera_config_t config;
config.ledc_channel = LEDC_CHANNEL_0;
config.ledc_timer = LEDC_TIMER_0;
config.pin_d0 = Y2_GPIO_NUM;
config.pin_d1 = Y3_GPIO_NUM;
config.pin_d2 = Y4_GPIO_NUM;
config.pin_d3 = Y5_GPIO_NUM;
config.pin_d4 = Y6_GPIO_NUM;
config.pin_d5 = Y7_GPIO_NUM;
config.pin_d6 = Y8_GPIO_NUM;
config.pin_d7 = Y9_GPIO_NUM;
config.pin_xclk = XCLK_GPIO_NUM;
config.pin_pclk = PCLK_GPIO_NUM;
config.pin_vsync = VSYNC_GPIO_NUM;
config.pin_href = HREF_GPIO_NUM;
config.pin_sscb_sda = SIOD_GPIO_NUM;
config.pin_sscb_scl = SIOC_GPIO_NUM;
config.pin_pwdn = PWDN_GPIO_NUM;
config.pin_reset = RESET_GPIO_NUM;
config.xclk_freq_hz = 20000000;
config.pixel_format = PIXFORMAT_JPEG;
config.frame_size = FRAMESIZE_SVGA;
config.jpeg_quality = 10;
config.fb_count = 2;
// Camera init
esp_err_t err = esp_camera_init(&config);
if (err != ESP_OK) {
return;
}
// Wi-Fi connection
const char *hostname = "SPEAR MKI";
WiFi.mode(WIFI_AP);
bool result = WiFi.softAP(hostname, "mjpeg-esp32", 1, 0);
WiFi.softAPConfig(apIP, apIP, IPAddress(255, 255, 255, 0));
if (!result)
{
return;
}
else
{
ip = WiFi.softAPIP();
}
// Start streaming web server
startCameraServer();
// Start tcp server on core 0
xTaskCreatePinnedToCore(
tcpCore, /* Function to implement the task */
"tcpServer", /* Name of the task */
10000, /* Stack size in words */
NULL, /* Task input parameter */
1, /* Priority of the task */
&tcpHandle, /* Task handle. */
0); /* Core where the task should run */
}
void loop() {
delay(10000);
}
----------------------------------------
Playstation gamepad PS3 over Bluetooth..
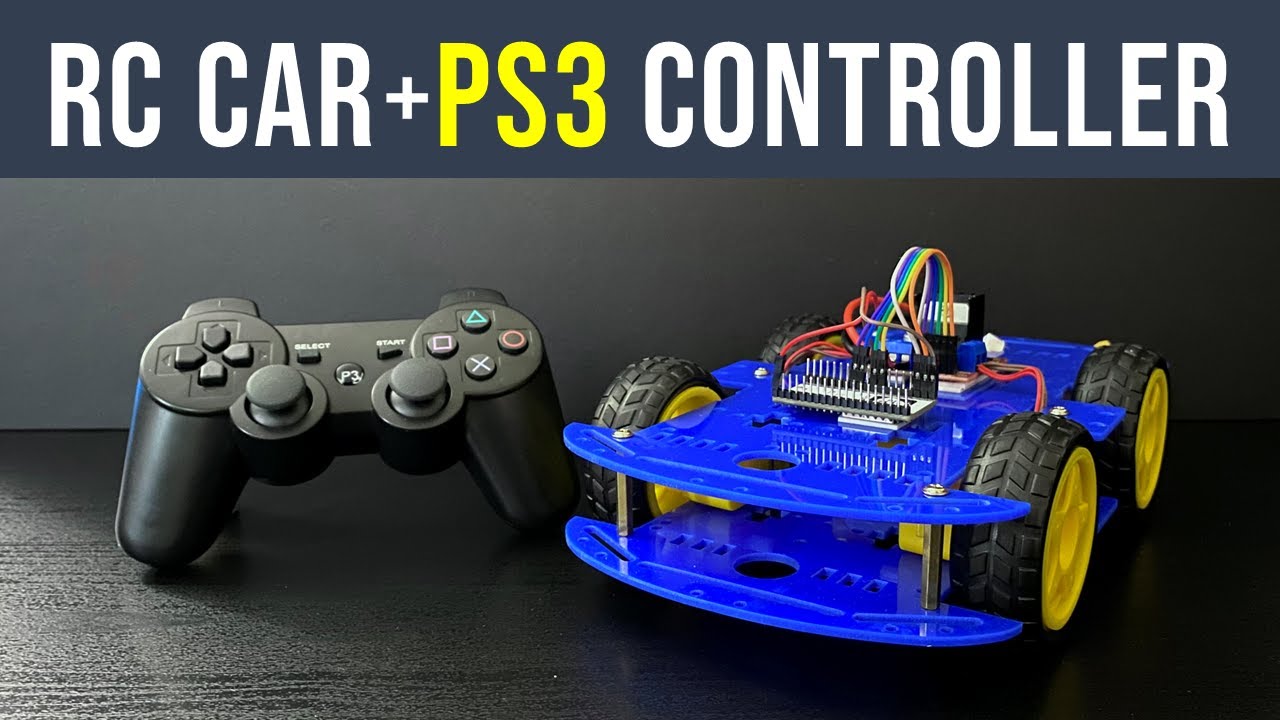
Code: Select all
#include <Ps3Controller.h>
int Flashlight = 4;
bool Flashlightstatus=false;
void notify()
{
//--- Digital cross/square/triangle/circle button events ---
if( Ps3.event.button_down.cross )
Serial.println("cross_on");
if( Ps3.event.button_up.cross )
Serial.println("cross_off");
if( Ps3.event.button_down.square )
Serial.println("square_on");
if( Ps3.event.button_up.square )
Serial.println("square_off");
if( Ps3.event.button_down.triangle )
Serial.println("triangle_on");
if( Ps3.event.button_up.triangle )
Serial.println("triangle_off");
if( Ps3.event.button_down.circle )
Serial.println("circle_on");
if( Ps3.event.button_up.circle )
Serial.println("circle_off");
//--------------- Digital D-pad button events --------------
if( Ps3.event.button_down.up ){
Serial.println("up_on");
}
if( Ps3.event.button_up.up ){
Serial.println("up_off");
}
if( Ps3.event.button_down.right ){
Serial.println("right_on");
}
if( Ps3.event.button_up.right ){
Serial.println("right_off");
}
if( Ps3.event.button_down.down ){
Serial.println("down_on");
}
if( Ps3.event.button_up.down ){
Serial.println("down_off");
}
if( Ps3.event.button_down.left ){
Serial.println("left_on");
}
if( Ps3.event.button_up.left ){
Serial.println("left_off");
}
//------------- Digital shoulder button events -------------
if( Ps3.event.button_down.l1 )
Serial.println("left1_on");
if( Ps3.event.button_up.l1 )
Serial.println("left1_off");
if( Ps3.event.button_down.r1 )
Serial.println("right1_on");
if( Ps3.event.button_up.r1 )
Serial.println("right1_off");
//-------------- Digital trigger button events -------------
if( Ps3.event.button_down.l2 )
Serial.println("left2_on");
if( Ps3.event.button_up.l2 )
Serial.println("left2_off");
if( Ps3.event.button_down.r2 )
Serial.println("right2_on");
if( Ps3.event.button_up.r2 )
Serial.println("right2_off");
//--------------- Digital stick button events --------------
if( Ps3.event.button_down.l3 )
Serial.println("left3_on");
if( Ps3.event.button_up.l3 )
Serial.println("left3_off");
if( Ps3.event.button_down.r3 )
Serial.println("right3_on");
if( Ps3.event.button_up.r3 )
Serial.println("right3_off");
//---------- Digital select/start/ps button events ---------
if( Ps3.event.button_down.select )
Serial.println("select_on");
if( Ps3.event.button_up.select )
Serial.println("select_off");
if( Ps3.event.button_down.start )
Serial.println("start_on");
if( Ps3.event.button_up.start )
Serial.println("start_off");
if( Ps3.event.button_down.ps ){
Flashlights();
Serial.println("ps_on");}
if( Ps3.event.button_up.ps ){
Serial.println("ps_off");}
//---------------- Analog stick value events ---------------
if( abs(Ps3.event.analog_changed.stick.lx) + abs(Ps3.event.analog_changed.stick.ly) > 2 ){
Serial.print("lsx"); Serial.println(Ps3.data.analog.stick.lx, DEC);
Serial.print("lsy"); Serial.println(Ps3.data.analog.stick.ly, DEC);
}
if( abs(Ps3.event.analog_changed.stick.rx) + abs(Ps3.event.analog_changed.stick.ry) > 2 ){
Serial.print("rsx"); Serial.println(Ps3.data.analog.stick.rx, DEC);
Serial.print("rsy"); Serial.println(Ps3.data.analog.stick.ry, DEC);
}
}
void onConnect(){
Serial.println("Connected.");
// Turn rumble on full intensity
Ps3.setRumble(100.0, 1000);
delay(500);
// Turn rumble on full intensity indefinitely
Ps3.setRumble(50.1000);
delay(500);
// Turn off rumble
Ps3.setRumble(0.0);
Flashlights();
delay(1000);
Flashlights();
}
void setup()
{
Serial.begin(115200);
Ps3.attach(notify);
Ps3.attachOnConnect(onConnect);
Ps3.begin("8c:ce:4e:88:47:ea");
Serial.println("Ready.");
pinMode(Flashlight, OUTPUT);
}
void loop(){
if(!Ps3.isConnected()){
return;
}
delay(100);
}
void Flashlights(void)
{
if (!Flashlightstatus){
digitalWrite(Flashlight,HIGH);
Flashlightstatus=true;}
else{
digitalWrite(Flashlight,LOW);
Flashlightstatus=false;}
}