I2C reading problem - PCF85063A
Posted: Sun May 23, 2021 4:59 pm
I'm stuck trying to interfacing a RTC (PCF85063A)
It seems bit 7 of every byte that it's been read is set to 1.
To debug I'm trying to write/read a value into device's RAM cell (reg 0x3)
In the below example it writes 0x05 and it reads 0x85.
Here are the oscilloscope views of write and read cycles:
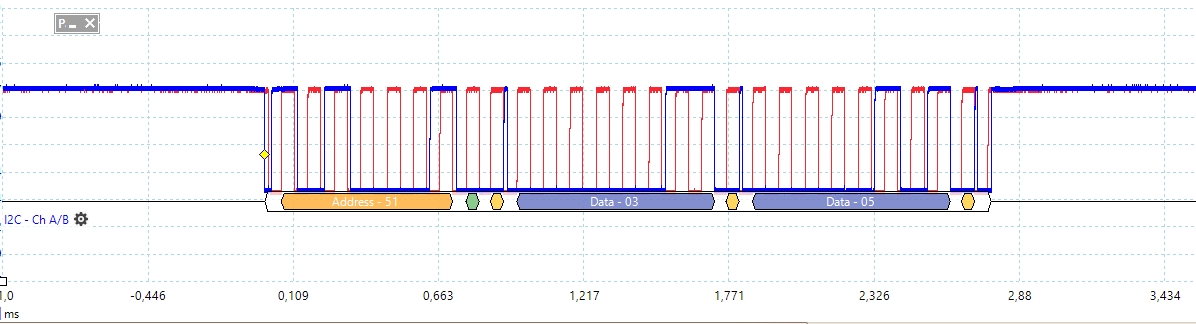
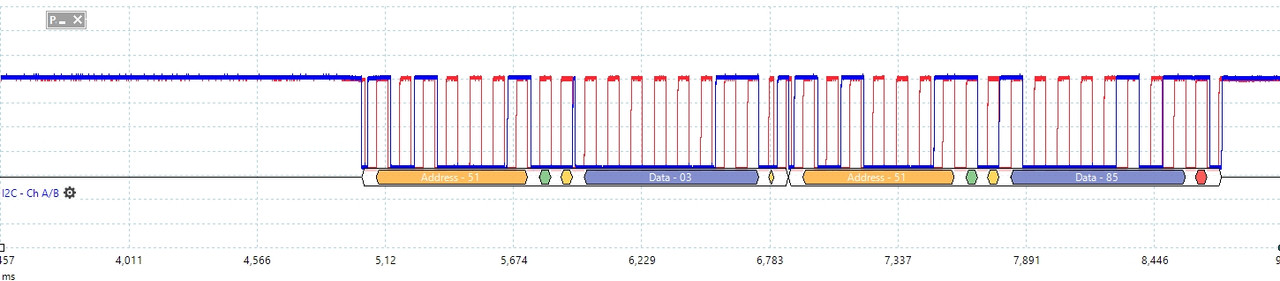
and here is the test code:
Any help is really appreciated, thanks...
It seems bit 7 of every byte that it's been read is set to 1.
To debug I'm trying to write/read a value into device's RAM cell (reg 0x3)
In the below example it writes 0x05 and it reads 0x85.
Here are the oscilloscope views of write and read cycles:
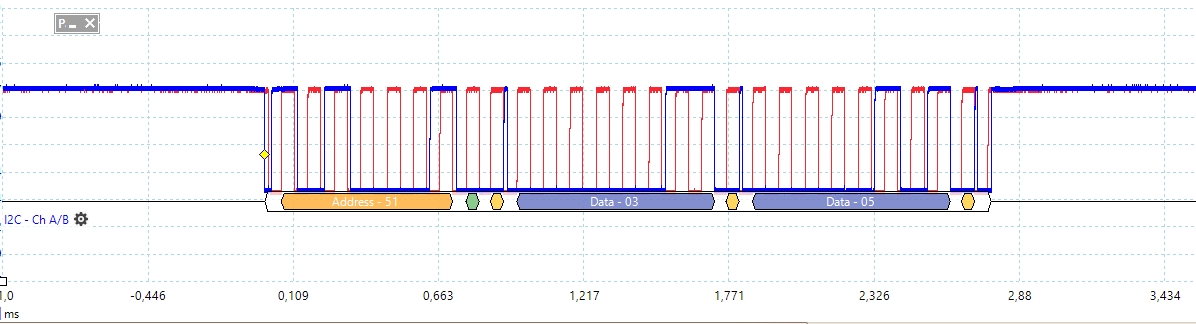
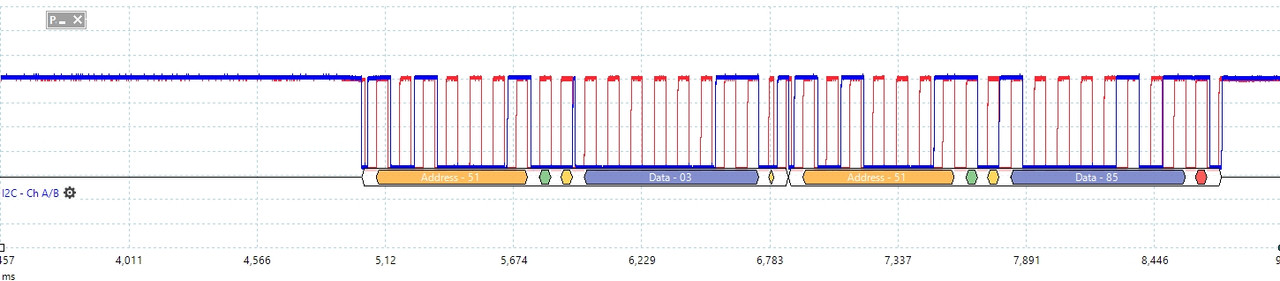
and here is the test code:
Code: Select all
#include "esp_log.h"
#include "driver/i2c.h"
#define ONBOARD_LEDRED 32
#define ONBOARD_LEDBLUE 33
static esp_err_t i2c_master_init(void)
{
i2c_config_t conf;
conf.mode = I2C_MODE_MASTER;
conf.sda_io_num = (gpio_num_t)21;
conf.scl_io_num = (gpio_num_t)22;
conf.sda_pullup_en = GPIO_PULLUP_DISABLE;
conf.scl_pullup_en = GPIO_PULLUP_DISABLE;
conf.master.clk_speed = 100000L;
i2c_param_config(I2C_NUM_0, &conf);
i2c_driver_install(I2C_NUM_0, I2C_MODE_MASTER, 0, 0, 0);
}
void writei2c(uint8_t reg,uint8_t value)
{
i2c_cmd_handle_t cmd = i2c_cmd_link_create();
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (0x51 << 1) | I2C_MASTER_WRITE, true);
i2c_master_write_byte(cmd, reg, I2C_MASTER_ACK);
i2c_master_write_byte(cmd, value, I2C_MASTER_ACK);
i2c_master_stop(cmd);
i2c_master_cmd_begin(I2C_NUM_0, cmd, 1000 / portTICK_RATE_MS);
i2c_cmd_link_delete(cmd);
}
uint8_t readi2c(uint8_t reg)
{
uint8_t rbyte;
i2c_cmd_handle_t cmd = i2c_cmd_link_create();
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (0x51 << 1) | I2C_MASTER_WRITE, 1);
i2c_master_write_byte(cmd, reg, 0);
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (0x51 << 1) | I2C_MASTER_READ, 1);
i2c_master_read_byte(cmd, &rbyte, (i2c_ack_type_t)1);
i2c_master_stop(cmd);
i2c_master_cmd_begin(I2C_NUM_0, cmd, 1000 / portTICK_RATE_MS);
i2c_cmd_link_delete(cmd);
return rbyte;
}
void setup() {
Serial.begin(115200);
i2c_master_init();
delay(500);
writei2c(REG_CTRL1,0b00000011);
delay(100);
pinMode(ONBOARD_LEDRED,OUTPUT);
pinMode(ONBOARD_LEDBLUE,OUTPUT);
}
void loop()
{
uint8_t datr=0;
writei2c(REG_RAM,5);
delay(2);
datr=readi2c(REG_RAM);
Serial.printf("Reg 0x3: %3u %3u",datw,datr);
delay(100);
}